Hi,
Here is the Procedure to load the sql data to excel with out writing lot of code
1)In Excel Click the Menu Data -> ImportExternalData -> NewDataBaseQuery
2)You will get the window Choose DataSource. Select New Datasource -> click OK
3)In Create New Data Source, give the name for the Datasource .Select the driver as SqlServer in the driver type.Click connect
4)Sql Server Login window opens ,Specify the Server Name.Click options Select the Database by default it will assign Master Database .Click Ok
5)Now the datasource will be connected.Select the table name to import your data in to excel.Click OK
6)Query Wizard Opens to select columns
7)Click the option .In the Table Options window there is a option to list only the tables,views, systemTables.Choose as per ur need.In the Owner select dbo.which excludes system tables.Click Next.Click Next
8)In query wizard- finish select the option view data or edit query in Microsoft Query.click Finish.
9)To execute your procedure click on File-> Execute Sql -> select Procedure
10)To import the data click File -> cancel and return to Microsoft excel
Thursday, January 29, 2009
Interface and abstract C#
Hi,
Here is the situation of using abstract vs interface . you may get some basic idea of using interface and abstract
Consider about the Content Management system we generalize the content as Articles,Blogs,Reviews
Interface Vs Abstract

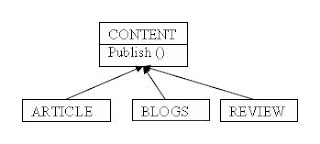
Now Consider Content Contains behavior named publish . If the Publish contain some default behavior then the option would be abstract. If the publish is derived and make their own implementation, then no need to implement in base .In this situation the option would be Interface.
If I made content class as Interface then it is difficult to make changes in base class because if I add new method or property in content interface then I have to implement new method in every derive class. This can be overcome in abstract class by creating new method not an abstract type.
In terms of speed abstract is fast then interface because interface requires extra in-direction.
Here is the situation of using abstract vs interface . you may get some basic idea of using interface and abstract
Consider about the Content Management system we generalize the content as Articles,Blogs,Reviews
Interface Vs Abstract

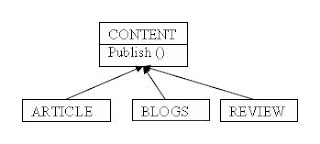
Now Consider Content Contains behavior named publish . If the Publish contain some default behavior then the option would be abstract. If the publish is derived and make their own implementation, then no need to implement in base .In this situation the option would be Interface.
If I made content class as Interface then it is difficult to make changes in base class because if I add new method or property in content interface then I have to implement new method in every derive class. This can be overcome in abstract class by creating new method not an abstract type.
In terms of speed abstract is fast then interface because interface requires extra in-direction.
Dynamic GridView C#
Hi,
Here is the code to create dynamic gridview with the styles applied
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["Sql"].
ConnectionString);
con.Open();
SqlCommand com = new SqlCommand("GetArticles", con);
com.CommandType = CommandType.StoredProcedure;
SqlDataAdapter ada = new SqlDataAdapter(com);
DataSet ds = new DataSet();
ada.Fill(ds);
for (int i = 0; i <>
{
if (ds.Tables[i].Rows.Count > 0)
{
GridView gvDynamicArticle = new GridView();
gvDynamicArticle.Width = Unit.Pixel(700);
gvDynamicArticle.BorderWidth = Unit.Pixel(0);
gvDynamicArticle.Caption = "
gvDynamicArticle.AutoGenerateColumns = false;
gvDynamicArticle.AlternatingRowStyle.BackColor = Color.Brown;
gvDynamicArticle.ShowFooter = true;
TemplateField tf = null;
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewTextTemplate("ArticleID", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewTextTemplate("ArticleID", DataControlRowType.DataRow);
tf.FooterTemplate = new DynamicGridViewTextTemplate(DataControlRowType.Footer, ds.Tables[i].Rows.Count);
gvDynamicArticle.Columns.Add(tf);
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewTextTemplate("Title", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewTextTemplate("Title", DataControlRowType.DataRow);
gvDynamicArticle.Columns.Add(tf);
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewTextTemplate("Description", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewTextTemplate("Description", DataControlRowType.DataRow);
gvDynamicArticle.Columns.Add(tf);
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewURLTemplate("Title", "URL", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewURLTemplate("Title", "URL", DataControlRowType.DataRow);
gvDynamicArticle.Columns.Add(tf);
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewTextTemplate("Author", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewTextTemplate("CreatedBy", DataControlRowType.DataRow);
gvDynamicArticle.Columns.Add(tf);
gvDynamicArticle.DataSource = ds.Tables[i];
gvDynamicArticle.DataBind();
phDynamicGridHolder.Controls.Add(gvDynamicArticle);
}
}
}
}
public class DynamicGridViewTextTemplate : ITemplate
{
string _ColName;
DataControlRowType _rowType;
int _Count;
public DynamicGridViewTextTemplate(string ColName, DataControlRowType RowType)
{
_ColName = ColName;
_rowType = RowType;
}
public DynamicGridViewTextTemplate(DataControlRowType RowType, int ArticleCount)
{
_rowType = RowType;
_Count = ArticleCount;
}
public void InstantiateIn(System.Web.UI.Control container)
{
switch (_rowType)
{
case DataControlRowType.Header:
Literal lc = new Literal();
lc.Text = "" + _ColName + "";
container.Controls.Add(lc);
break;
case DataControlRowType.DataRow:
Label lbl = new Label();
lbl.DataBinding += new EventHandler(this.lbl_DataBind);
container.Controls.Add(lbl);
break;
case DataControlRowType.Footer:
Literal lc1 = new Literal();
lc1.Text = " ";
container.Controls.Add(lc1);
break;
default:
break;
}
}
private void lbl_DataBind(Object sender, EventArgs e)
{
Label lbl = (Label)sender;
GridViewRow row = (GridViewRow)lbl.NamingContainer;
lbl.Text =DataBinder.Eval(row.DataItem, _ColName).ToString();
}
}
public class DynamicGridViewURLTemplate : ITemplate
{
string _ColNameText;
string _ColNameURL;
DataControlRowType _rowType;
public DynamicGridViewURLTemplate(string ColNameText, string ColNameURL, DataControlRowType RowType)
{
_ColNameText = ColNameText;
_rowType = RowType;
_ColNameURL = ColNameURL;
}
public void InstantiateIn(System.Web.UI.Control container)
{
switch (_rowType)
{
case DataControlRowType.Header:
Literal lc = new Literal();
lc.Text = "" + _ColNameURL + "";
container.Controls.Add(lc);
break;
case DataControlRowType.DataRow:
HyperLink hpl = new HyperLink();
hpl.Target = "_blank";
hpl.DataBinding += new EventHandler(this.hpl_DataBind);
container.Controls.Add(hpl);
break;
default:
break;
}
}
private void hpl_DataBind(Object sender, EventArgs e)
{
HyperLink hpl = (HyperLink)sender;
GridViewRow row = (GridViewRow)hpl.NamingContainer;
hpl.NavigateUrl = DataBinder.Eval(row.DataItem, _ColNameURL).ToString();
hpl.Text = "";
}
Here is the code to create dynamic gridview with the styles applied
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["Sql"].
ConnectionString);
con.Open();
SqlCommand com = new SqlCommand("GetArticles", con);
com.CommandType = CommandType.StoredProcedure;
SqlDataAdapter ada = new SqlDataAdapter(com);
DataSet ds = new DataSet();
ada.Fill(ds);
for (int i = 0; i <>
{
if (ds.Tables[i].Rows.Count > 0)
{
GridView gvDynamicArticle = new GridView();
gvDynamicArticle.Width = Unit.Pixel(700);
gvDynamicArticle.BorderWidth = Unit.Pixel(0);
gvDynamicArticle.Caption = "
" + ds.Tables[i].Rows[0]["Category"].ToString() + " Articles
";gvDynamicArticle.AutoGenerateColumns = false;
gvDynamicArticle.AlternatingRowStyle.BackColor = Color.Brown;
gvDynamicArticle.ShowFooter = true;
TemplateField tf = null;
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewTextTemplate("ArticleID", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewTextTemplate("ArticleID", DataControlRowType.DataRow);
tf.FooterTemplate = new DynamicGridViewTextTemplate(DataControlRowType.Footer, ds.Tables[i].Rows.Count);
gvDynamicArticle.Columns.Add(tf);
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewTextTemplate("Title", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewTextTemplate("Title", DataControlRowType.DataRow);
gvDynamicArticle.Columns.Add(tf);
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewTextTemplate("Description", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewTextTemplate("Description", DataControlRowType.DataRow);
gvDynamicArticle.Columns.Add(tf);
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewURLTemplate("Title", "URL", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewURLTemplate("Title", "URL", DataControlRowType.DataRow);
gvDynamicArticle.Columns.Add(tf);
tf = new TemplateField();
tf.HeaderTemplate = new DynamicGridViewTextTemplate("Author", DataControlRowType.Header);
tf.ItemTemplate = new DynamicGridViewTextTemplate("CreatedBy", DataControlRowType.DataRow);
gvDynamicArticle.Columns.Add(tf);
gvDynamicArticle.DataSource = ds.Tables[i];
gvDynamicArticle.DataBind();
phDynamicGridHolder.Controls.Add(gvDynamicArticle);
}
}
}
}
public class DynamicGridViewTextTemplate : ITemplate
{
string _ColName;
DataControlRowType _rowType;
int _Count;
public DynamicGridViewTextTemplate(string ColName, DataControlRowType RowType)
{
_ColName = ColName;
_rowType = RowType;
}
public DynamicGridViewTextTemplate(DataControlRowType RowType, int ArticleCount)
{
_rowType = RowType;
_Count = ArticleCount;
}
public void InstantiateIn(System.Web.UI.Control container)
{
switch (_rowType)
{
case DataControlRowType.Header:
Literal lc = new Literal();
lc.Text = "" + _ColName + "";
container.Controls.Add(lc);
break;
case DataControlRowType.DataRow:
Label lbl = new Label();
lbl.DataBinding += new EventHandler(this.lbl_DataBind);
container.Controls.Add(lbl);
break;
case DataControlRowType.Footer:
Literal lc1 = new Literal();
lc1.Text = " ";
container.Controls.Add(lc1);
break;
default:
break;
}
}
private void lbl_DataBind(Object sender, EventArgs e)
{
Label lbl = (Label)sender;
GridViewRow row = (GridViewRow)lbl.NamingContainer;
lbl.Text =DataBinder.Eval(row.DataItem, _ColName).ToString();
}
}
public class DynamicGridViewURLTemplate : ITemplate
{
string _ColNameText;
string _ColNameURL;
DataControlRowType _rowType;
public DynamicGridViewURLTemplate(string ColNameText, string ColNameURL, DataControlRowType RowType)
{
_ColNameText = ColNameText;
_rowType = RowType;
_ColNameURL = ColNameURL;
}
public void InstantiateIn(System.Web.UI.Control container)
{
switch (_rowType)
{
case DataControlRowType.Header:
Literal lc = new Literal();
lc.Text = "" + _ColNameURL + "";
container.Controls.Add(lc);
break;
case DataControlRowType.DataRow:
HyperLink hpl = new HyperLink();
hpl.Target = "_blank";
hpl.DataBinding += new EventHandler(this.hpl_DataBind);
container.Controls.Add(hpl);
break;
default:
break;
}
}
private void hpl_DataBind(Object sender, EventArgs e)
{
HyperLink hpl = (HyperLink)sender;
GridViewRow row = (GridViewRow)hpl.NamingContainer;
hpl.NavigateUrl = DataBinder.Eval(row.DataItem, _ColNameURL).ToString();
hpl.Text = "
" + DataBinder.Eval(row.DataItem, _ColNameText).ToString() + "
}
Subscribe to:
Posts (Atom)